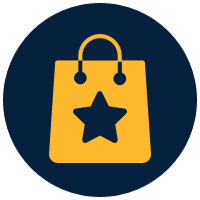
Unlimited AI-Powered Learning
Level up your skills! Get 34% off Cosmo+ with code HOLIDAY24. Limited time only!
This comprehensive course is specially designed to impart a fundamental and advanced understanding of sorting and searching algorithms. The course will guide you through different types and functionalities of these algorithms, their complexity analysis, and practical application in resolving complex programming problems.