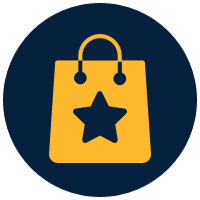
Unlimited AI-Powered Learning
Level up your skills! Get 34% off Cosmo+ with code HOLIDAY24. Limited time only!
This is a comprehensive course curated to convey fundamental and advanced understanding of sorting and searching algorithms. The course guides through different types and functionality of these algorithms, their complexity analysis, and the practical application in solving complex programming problems.