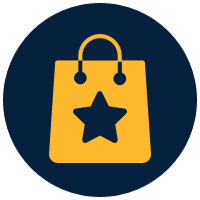
Unlimited AI-Powered Learning
Level up your skills! Get 34% off Cosmo+ with code HOLIDAY24. Limited time only!
Explore and revise fundamental software design patterns and their applications in Python, essential for structuring flexible and robust software solutions.