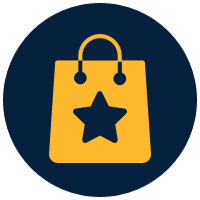
Unlimited AI-Powered Learning
Level up your skills! Get 34% off Cosmo+ with code HOLIDAY24. Limited time only!
Dive into four engaging units that take you from basic user authentication to mastering JWT tokens. Learn to secure your endpoints, handle tokens like a pro, and build robust, authenticated APIs effortlessly.