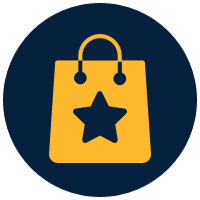
Unlimited AI-Powered Learning
Level up your skills! Get 34% off Cosmo+ with code HOLIDAY24. Limited time only!
Strengthen your understanding and application of hashmap data structures with a focus on counting and aggregation tasks. This course will deepen your comprehension of efficient data access and manipulation using hashmaps.