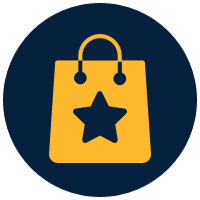
Unlimited AI-Powered Learning
Level up your skills! Get 34% off Cosmo+ with code HOLIDAY24. Limited time only!
Focusing on data manipulation operations, this course teaches how to perform data projection, filtering, and aggregation using C++ without needing advanced external libraries. You will learn how to use both procedural and functional programming techniques when applying filtering and aggregation on the data stream.