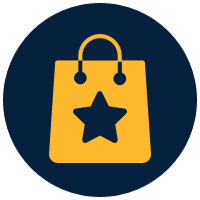
Unlimited AI-Powered Learning
Level up your skills! Get 34% off Cosmo+ with code HOLIDAY24. Limited time only!
Structural design patterns manage object composition and relationships, improving flexibility and scalability of systems. This course covers key structural patterns like Adapter, Composite, and Decorator, demonstrating their application in GUI libraries, file systems, and more, to solve real-world problems.