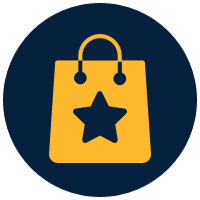
Unlimited AI-Powered Learning
Level up your skills! Get 34% off Cosmo+ with code HOLIDAY24. Limited time only!
Learn how to build complex systems using Object-oriented programming princinples. Master Inheritance and Polymorphism. Learn to organize your code structure to build maintainable programs.