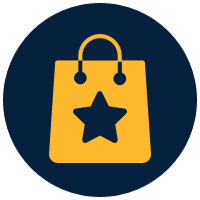
Unlimited AI-Powered Learning
Level up your skills! Get 34% off Cosmo+ with code HOLIDAY24. Limited time only!
This course is designed to demonstrate the representation of a graph using adjacency graphs and adjacency matrices in Python. A core part of the course is dedicated to implementing and utilizing BFS and DFS algorithms in graphs. Explore the comprehensive use of graph data structures in solving intricate interview-based algorithmic problems.