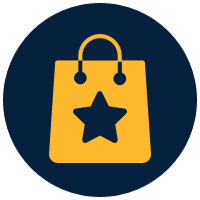
Unlimited AI-Powered Learning
Level up your skills! Get 34% off Cosmo+ with code HOLIDAY24. Limited time only!
This course will help you dive deep into the world of function constructs in Python. You will revisit the basics of functions, explore advanced topics like lambda expressions and recursion, and master techniques essential for efficient and effective function usage within your programs.