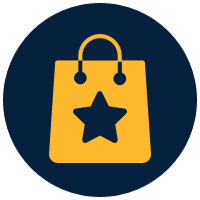
Unlimited AI-Powered Learning
Level up your skills! Get 34% off Cosmo+ with code HOLIDAY24. Limited time only!
Dive into the key algorithms involving Maps, Sets, and two-pointer techniques. This course will enhance your skills in optimizing data structures and problem-solving methods.