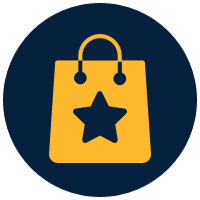
Unlimited AI-Powered Learning
Level up your skills! Get 34% off Cosmo+ with code HOLIDAY24. Limited time only!
Explore Java's native data structures like arrays, ArrayLists, and HashMaps. This course strengthens your skills to handle varying data sizes and complexities in coding.