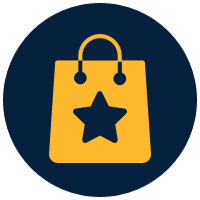
Unlimited AI-Powered Learning
Level up your skills! Get 34% off Cosmo+ with code HOLIDAY24. Limited time only!
Traverse through fascinating C++ data structures such as arrays, vectors, strings, sets and maps. Learn to manage and manipulate these structures effectively and use their advantages to boost your code.