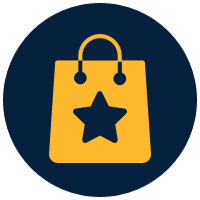
Overview
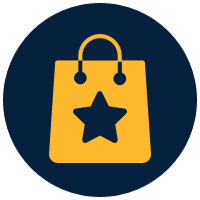
Master the fundamentals of multithreading in C++ with this course path. Learn how to create threads, synchronize them, how to create concurrent data structures. Understand how to avoid common multithreading pitfalls. Get hands-on experience with real-world examples and exercises.
Syllabus
- Introduction to C++ Concurrency
- This course provides an introduction to concurrency in C++. It covers fundamental concepts, including threads, the C++ Memory Model, and basic synchronization techniques. Students will learn how to create and manage threads, understand data sharing between threads using primitive approaches, and grasp the importance of synchronization to prevent concurrency issues like race conditions.
- Concurrency Essentials in C++
- This course delves into the core concepts of C++ concurrency. It covers synchronization mechanisms, including atomic operations and mutexes. Students will learn about the C++ Memory Model, inter-thread communication using condition variables, lock-free programming with atomic variables, and how to avoid common concurrency issues like deadlocks and race conditions.
- Practice Tasks for Concurrency Essentials
- This practice-based course reinforces the concepts learned in the previous course `Concurrency Essentials`. It includes real-life tasks that focus on synchronization mechanisms, lock-free programming, deadlock prevention, and inter-thread communication. Each unit provides a practical scenario where students can apply their knowledge to solve concurrency challenges.
- Lock-Based Concurrent Data Structures
- This course focuses on lock-based data structures in C++. It covers synchronization mechanisms like mutexes and condition variables to implement thread-safe data structures. Students will learn how to create lock-based concurrent data structures like stacks, queues, and lists, and understand the benefits and challenges of using locks for synchronization.
- Lock-Free Concurrent Data Structures
- This course focuses on lock-free data structures in C++. It covers atomic operations, memory ordering, and lock-free algorithms. Students will learn how to implement and use lock-free data structures like queues, stacks, maps, and sets, as well as understand the benefits and challenges of lock-free programming.
Courses
-
This course provides an introduction to concurrency in C++. It covers fundamental concepts, including threads, the C++ Memory Model, and basic synchronization techniques. Students will learn how to create and manage threads, understand data sharing between threads using primitive approaches, and grasp the importance of synchronization to prevent concurrency issues like race conditions.
-
This course delves into the core concepts of C++ concurrency. It covers synchronization mechanisms, including atomic operations and mutexes. Students will learn about the C++ Memory Model, inter-thread communication using condition variables, lock-free programming with atomic variables, and how to avoid common concurrency issues like deadlocks and race conditions.
-
This practice-based course reinforces the concepts learned in the previous course `Concurrency Essentials`. It includes real-life tasks that focus on synchronization mechanisms, lock-free programming, deadlock prevention, and inter-thread communication. Each unit provides a practical scenario where students can apply their knowledge to solve concurrency challenges.
-
This course focuses on lock-based data structures in C++. It covers synchronization mechanisms like mutexes and condition variables to implement thread-safe data structures. Students will learn how to create lock-based concurrent data structures like stacks, queues, and lists, and understand the benefits and challenges of using locks for synchronization.
-
This course focuses on lock-free data structures in C++. It covers atomic operations, memory ordering, and lock-free algorithms. Students will learn how to implement and use lock-free data structures like queues, stacks, maps, and sets, as well as understand the benefits and challenges of lock-free programming.