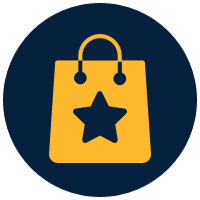
Unlimited AI-Powered Learning
Level up your skills! Get 34% off Cosmo+ with code HOLIDAY24. Limited time only!
This course provides an introduction and basic understanding of the Go programming language. Participants will consolidate their understanding of key Go Syntax, scripting, and problem-solving functionalities.