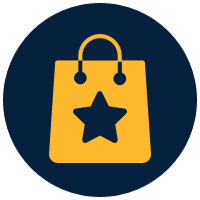
Unlimited AI-Powered Learning
Level up your skills! Get 34% off Cosmo+ with code HOLIDAY24. Limited time only!
In this course, you'll delve deeply into algorithms and data structures, which are key topics in technical interviews. You'll tackle problems involving linked lists, binary trees, dynamic programming, and graph algorithms. Understanding these advanced topics will equip you with the tools needed to solve complex problems efficiently.