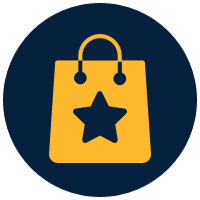
Unlimited AI-Powered Learning
Level up your skills! Get 34% off Cosmo+ with code HOLIDAY24. Limited time only!
Start your coding interview preparation with easy-level C++ problems. This course will help you build a solid foundation by solving common problems like reversing a string, finding the maximum number in a list, and checking for prime numbers. These exercises will enhance your problem-solving skills and boost your confidence for the coding interviews.