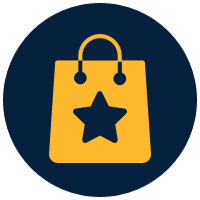
Unlimited AI-Powered Learning
Level up your skills! Get 34% off Cosmo+ with code HOLIDAY24. Limited time only!
Begin your programming journey with C++ by laying a solid foundation of basic syntax, data types and control structures. This course is practically oriented, enabling you to immediately put new skills into practice with engaging coding challenges.