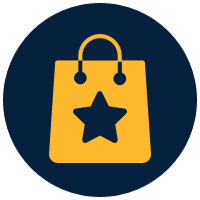
Unlimited AI-Powered Learning
Level up your skills! Get 34% off Cosmo+ with code HOLIDAY24. Limited time only!
Explore advanced text classification techniques, including ensemble methods and deep learning, to enhance model performance using Python and TensorFlow.