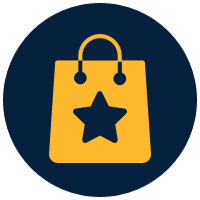
Unlimited AI-Powered Learning
Level up your skills! Get 34% off Cosmo+ with code HOLIDAY24. Limited time only!
This advanced course will introduce you to the concept of monads in C++ and teach you how to implement and compose them. You will explore the design patterns functors, monads, and monad transformers, along with practical usage scenarios.