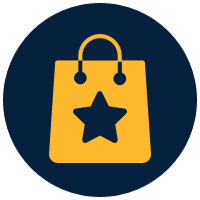
Unlimited AI-Powered Learning
Level up your skills! Get 34% off Cosmo+ with code HOLIDAY24. Limited time only!
This advanced course furthers into the understanding and application of Stacks and Queues in Java. It explicates the inner workings, implementation, and complexities of these structures, with their effectiveness for resolving interview-focused algorithmic coding conundrums.